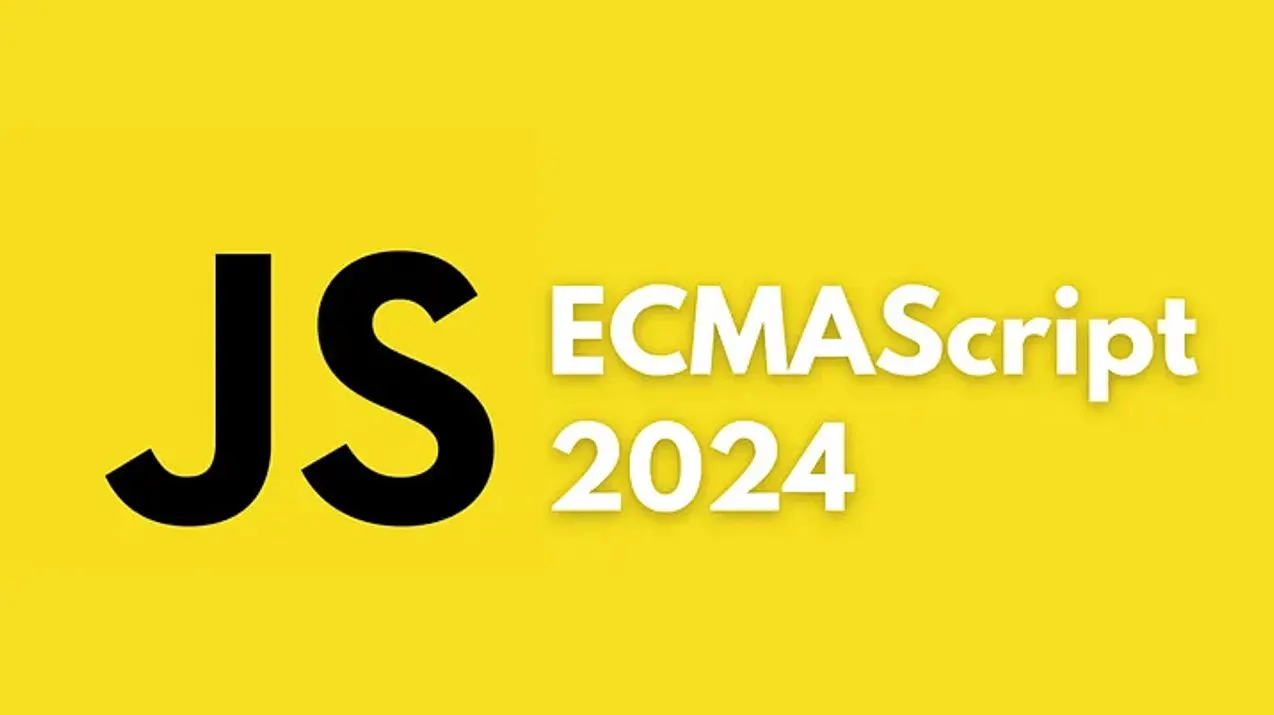
New Features of JavaScript ES2024
With the release of JavaScript ES2024, developers worldwide are now equipped with a host of new features designed to improve code efficiency, readability, and functionality. These advancements highlight JavaScript’s continued evolution as the cornerstone of web development. In this article, we explore three major updates in ES2024: enhanced array methods, asynchronous iterator improvements, and the introduction of record and tuple types. Each feature promises to streamline workflows and expand the language’s capabilities. Let’s dive into these updates in detail.
Enhanced Array Methods
JavaScript ES2024 introduces new array methods that simplify complex data manipulation tasks. These methods build on the already robust array functionalities, making operations like filtering, mapping, and reducing even more intuitive.
Arrays have always been a fundamental part of JavaScript, and ES2024 takes their utility to the next level. Developers often need to process large datasets, which involves filtering data, transforming it, and then reducing it into a meaningful result. The new methods introduced in ES2024 allow combining these steps into single, optimised operations, saving both time and computational resources. This also means fewer lines of code, which results in better readability and maintainability of scripts.
These methods reflect JavaScript’s dedication to addressing common developer challenges. Whether it’s handling edge cases or reducing the performance impact of iterative loops, the new updates bring improvements grounded in real-world application needs. The enhancement of array methods ensures developers can write expressive, concise, and efficient code without compromising on performance.
Key Enhancements in Array Methods
One of the standout additions is the `filterMap` method. This method combines filtering and mapping in a single operation, reducing the need for multiple iterations over an array. For instance, developers can now filter out unwanted elements and transform the remaining ones with a concise syntax:
const result = array.filterMap((item) => condition(item) ? transform(item) : null);
Another improvement is the introduction of `findLastIndex`, allowing developers to locate the last occurrence of an element matching a specific condition. This complements the existing `find` and `findIndex` methods, providing a more comprehensive toolkit for array handling. Together, these features are expected to significantly reduce boilerplate code and improve overall coding efficiency. The seamless integration of these methods underscores ES2024’s emphasis on practical improvements for developers.
Asynchronous Iterator Improvements
Asynchronous programming has become a staple in modern JavaScript, and ES2024 builds on this with improvements to asynchronous iterators. These updates aim to make handling streams of data more efficient and intuitive.
The demand for asynchronous programming capabilities has grown alongside the rise of APIs and real-time data streams. JavaScript’s async iterators have been a critical tool for managing these streams, and ES2024 takes this functionality even further. The improved iterators allow developers to create cleaner and more readable code when working with large-scale, dynamic datasets. Whether you’re handling user input, live updates, or server communications, these enhancements simplify the coding experience.
This is particularly beneficial for projects that involve heavy use of asynchronous operations, such as web scraping, data analysis pipelines, and online gaming systems. The new features ensure that developers can handle multiple streams without resorting to complex nested structures or custom utilities, reducing the overall risk of errors.
New Features in Async Iterators
One notable addition is the `asyncFlatten` function, which enables developers to process nested asynchronous iterables seamlessly. This feature eliminates the need for cumbersome nesting logic, simplifying codebases that rely heavily on async operations. For example:
for await (const item of asyncFlatten(asyncIterable)) { console.log(item); }
Additionally, ES2024 introduces `forEachAsync`, which extends the `forEach` concept to asynchronous iterables. This allows developers to execute asynchronous functions on each element without manually handling promises. These updates represent a significant leap forward in asynchronous data management. With these tools, developers can focus more on building robust applications and less on writing repetitive boilerplate code.
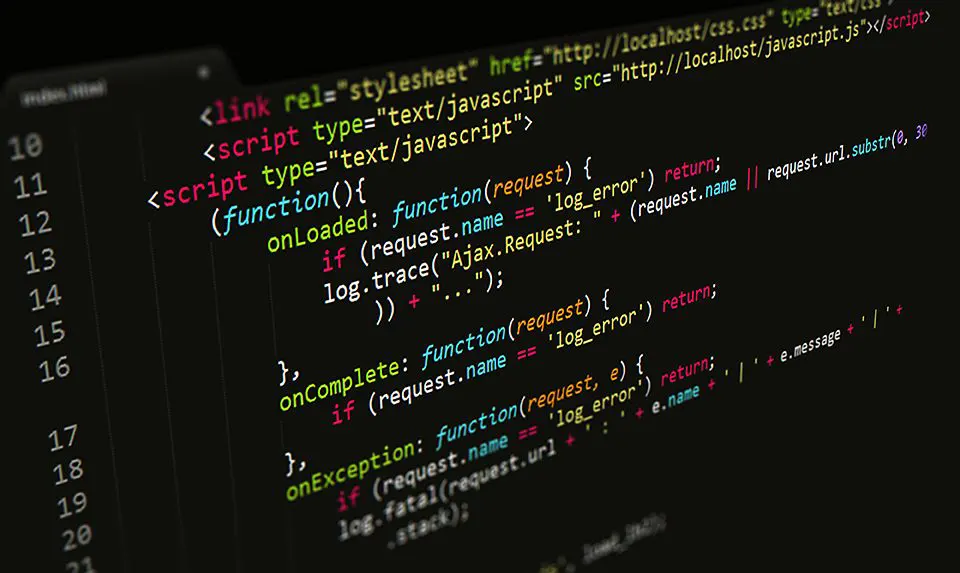
Introduction of Record and Tuple Types
One of the most anticipated features of ES2024 is the inclusion of record and tuple types. These immutable data structures provide a robust alternative to traditional objects and arrays, ensuring data integrity and simplifying comparisons.
Immutability has long been a sought-after characteristic in programming, particularly in functional programming paradigms. Records and tuples address this demand by offering deeply immutable structures that integrate seamlessly into JavaScript. By preventing unintended modifications, these types reduce bugs related to state changes and improve application stability. This is especially critical in large-scale applications where data consistency is paramount.
These features also encourage best practices for developers. When working in collaborative environments, immutability ensures that data remains reliable regardless of how many modules interact with it. This greatly simplifies debugging and testing, as developers can rely on the predictability of data structures.
Benefits of Records and Tuples
Records are key-value pairs similar to objects but are deeply immutable. This makes them ideal for scenarios requiring constant data. Tuples, on the other hand, are ordered lists of values, similar to arrays but with guaranteed immutability. These features make comparing complex structures effortless, as their equality is determined by value rather than reference.
For example, consider the following:
const record = #{ key: 'value' }; const tuple = #[1, 2, 3];
Such constructs enhance code predictability and facilitate debugging, especially in applications where immutability is crucial. With these updates, JavaScript ES2024 sets a new standard for efficiency and reliability in software development. Developers can now write cleaner, more maintainable code while ensuring that their applications remain robust and performant under various conditions.